On October 6th afternoon I got a gig offer from a South Korean (his username was fred82162, I will call him Fred in this article) about adding Google search bar beside top menu on his WordPress website. He gave me screenshots of his website indicating what must be changed and where it should be placed.
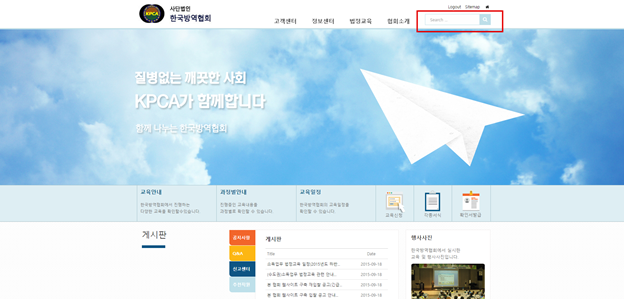
The red rectangle in the above screenshot points to a search bar beside top menu bar. It must be replaced with Google search bar. Initially this looked like an easy task so I charged him very less. But during the work it came to my mind that I had to work hard to get this done. It felt like I should have charged more but then couldn’t do anything.
Fred said the search bar was just a normal WordPress search bar, to display it at top besides menu he used Search box on Navigation Menu plugin. Instead the plugin he wants to use is WP Google Search plugin. Fred said that “whatever customization you are doing should not change the design, only function has to change”. I said “agreed!!”
He gave me all the details required for the work to be done. His website is www.ikpca.com. Fred had already installed WP Google Search plugin on his website and is ready to be used. Navigating through his WordPress Dashboard I have seen that he is using Avada WordPress theme.
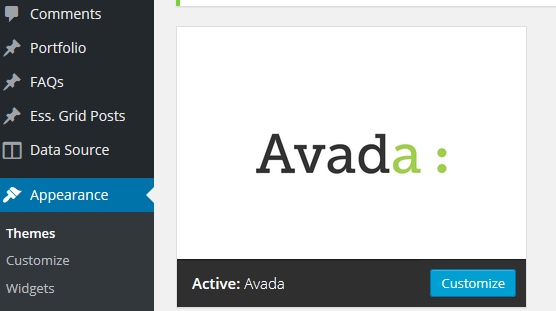
As I looked at header.php, it was clear that this code was very hard to understand. It was like full of custom theme framework. Initially I was looking at header.php for search box code. After some time searching here and there I found the searchform.php file. It gave me a big relief.
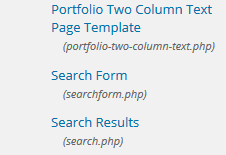
Fred told me that he used plugin to display search box at top. So I opened the Search box on Navigation menu plugin in editor. The code was very tiny for this plugin (see the code below).
add_filter('wp_nav_menu_items','add_search_box', 10, 2);
function add_search_box($items, $args) {
ob_start();
get_search_form();
$searchform = ob_get_contents();
ob_end_clean();
$items .= '<li>' . $searchform . '</li>';
return $items;
}
As I am familiar with WordPress plugin code I quickly understood what is going on in this code. Getting the contents of searchform.php and displaying it in list tags. So I thought why not display the Google search bar using the shortcode here itself. WP Google Search has shortcode for display purpose. Using the same Search box on Navigation menu plugin I displayed the Google search bar using the shortcode the plugin provided. The code looked like this.
add_filter('wp_nav_menu_items','add_search_box', 10, 2);
function add_search_box($items, $args) {
ob_start();
$items .='<li>' .do_shortcode('[wp_google_searchbox]'). '</li>';
echo $items;
$output = ob_get_clean();
return $output;
}
You can display shortcode using echo do_shortcode(‘[wp_google_searchbox]’); statement in WordPress. Echoing this statement in the plugin replaced all the menu items with Google search bar. It destroyed all the design. The shortcode value has to be returned in plugin to make it work normal. So I came across this page. On this page they were using somewhat similar code that I am seeing in this plugin editor. I changed it where ever needed and got a working code out of it. The code above displays the Google search bar right beside the top menu. I changed CSS styling codes and made it look like this finally.
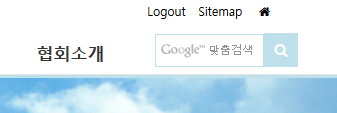
Google search bar that I placed works fine on this website. Fred was very happy to see this. He gave me 5 star rating and paid me.

If you have any WordPress issue offer me a gig here on this page. I will let you know if I can do it or not.
Read More...